Pie Chart: Using C/C++ and graphics
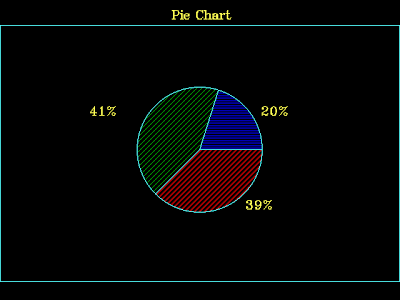
Hey guys, its very simple illustration of pie chart but it is not exactly how it works. In next post I will post how to take input from user and then generate the pie chart and bar graph. Here is the screen shot of the output. Pie-chart using C/C++ and Graphics Here's the code, enjoy coding.. /* Programmer : Ashok Kumar Shrestha (ak007) Program details : Pie chart */ #include<stdio.h> #include<graphics.h> #include<conio.h> int main() { int gd = DETECT, gm, midx, midy; initgraph(&gd,&gm,"..\\bgi"); setcolor(11); rectangle(0,40,639,450); settextstyle(1,0,2); midx = getmaxx()/2; midy = getmaxy()/2; setfillstyle(2,1); pieslice(midx, midy, 0, 72, 100); setfillstyle(3,2); pieslice(midx, midy, 72, 225, 100); setfillstyle(4,4); pieslice(midx, midy, 225, ...